Note
Go to the end to download the full example code. or to run this example in your browser via Binder
Load and Plot from a File#
Read a dataset from a known file type.
We try to make loading a mesh as easy as possible - if your data is in one
of the many supported file formats, simply use pyvista.read()
to
load your spatially referenced dataset into a PyVista mesh object.
The following code block uses a built-in example file and displays an airplane mesh.
import pyvista as pv
from pyvista import examples
help(pv.read)
Help on function read in module pyvista.core.utilities.fileio:
read(filename: 'PathStrSeq', force_ext: 'str | None' = None, file_format: 'str | None' = None, progress_bar: 'bool' = False) -> 'DataObject'
Read any file type supported by ``vtk`` or ``meshio``.
Automatically determines the correct reader to use then wraps the
corresponding mesh as a pyvista object. Attempts native ``vtk``
readers first then tries to use ``meshio``. :py:mod:`Pickled<pickle>`
meshes (``'.pkl'`` or ``'.pickle'``) are also supported.
See :func:`pyvista.get_reader` for list of vtk formats supported.
.. note::
See https://github.com/nschloe/meshio for formats supported by
``meshio``. Be sure to install ``meshio`` with ``pip install
meshio`` if you wish to use it.
.. versionadded:: 0.45
Support reading pickled meshes.
.. warning::
The pickle module is not secure. Only read pickled mesh files
(``'.pkl'`` or ``'.pickle'``) you trust. See :py:mod:`pickle`
for details.
See Also
--------
pyvista.DataObject.save
Save a mesh to file.
Parameters
----------
filename : str, Path, Sequence[str | Path]
The string path to the file to read. If a list of files is
given, a :class:`pyvista.MultiBlock` dataset is returned with
each file being a separate block in the dataset.
force_ext : str, optional
If specified, the reader will be chosen by an extension which
is different to its actual extension. For example, ``'.vts'``,
``'.vtu'``.
file_format : str, optional
Format of file to read with meshio.
progress_bar : bool, default: False
Optionally show a progress bar. Ignored when using ``meshio``.
Returns
-------
pyvista.DataSet
Wrapped PyVista dataset.
Examples
--------
Load an example mesh.
>>> import pyvista as pv
>>> from pyvista import examples
>>> mesh = pv.read(examples.antfile)
>>> mesh.plot(cpos='xz')
Load a vtk file.
>>> mesh = pv.read('my_mesh.vtk') # doctest:+SKIP
Load a meshio file.
>>> mesh = pv.read('mesh.obj') # doctest:+SKIP
Load a pickled mesh file.
>>> mesh = pv.read('mesh.pkl') # doctest:+SKIP
PyVista supports a wide variety of file formats. The supported file extensions are listed in an internal function:
Help on function get_reader in module pyvista.core.utilities.reader:
get_reader(filename, force_ext=None)
Get a reader for fine-grained control of reading data files.
Supported file types and Readers:
+----------------+---------------------------------------------+
| File Extension | Class |
+================+=============================================+
| ``.bmp`` | :class:`pyvista.BMPReader` |
+----------------+---------------------------------------------+
| ``.cas`` | :class:`pyvista.FluentReader` |
+----------------+---------------------------------------------+
| ``.case`` | :class:`pyvista.EnSightReader` |
+----------------+---------------------------------------------+
| ``.cgns`` | :class:`pyvista.CGNSReader` |
+----------------+---------------------------------------------+
| ``.cube`` | :class:`pyvista.GaussianCubeReader` |
+----------------+---------------------------------------------+
| ``.dat`` | :class:`pyvista.TecplotReader` |
+----------------+---------------------------------------------+
| ``.dcm`` | :class:`pyvista.DICOMReader` |
+----------------+---------------------------------------------+
| ``.dem`` | :class:`pyvista.DEMReader` |
+----------------+---------------------------------------------+
| ``.e`` | :class:`pyvista.ExodusIIReader` |
+----------------+---------------------------------------------+
| ``.exo`` | :class:`pyvista.ExodusIIReader` |
+----------------+---------------------------------------------+
| ``.exii`` | :class:`pyvista.ExodusIIReader` |
+----------------+---------------------------------------------+
| ``.ex2`` | :class:`pyvista.ExodusIIReader` |
+----------------+---------------------------------------------+
| ``.facet`` | :class:`pyvista.FacetReader` |
+----------------+---------------------------------------------+
| ``.foam`` | :class:`pyvista.POpenFOAMReader` |
+----------------+---------------------------------------------+
| ``.g`` | :class:`pyvista.BYUReader` |
+----------------+---------------------------------------------+
| ``.gif`` | :class:`pyvista.GIFReader` |
+----------------+---------------------------------------------+
| ``.glb`` | :class:`pyvista.GLTFReader` |
+----------------+---------------------------------------------+
| ``.gltf`` | :class:`pyvista.GLTFReader` |
+----------------+---------------------------------------------+
| ``.hdf`` | :class:`pyvista.HDFReader` |
+----------------+---------------------------------------------+
| ``.img`` | :class:`pyvista.DICOMReader` |
+----------------+---------------------------------------------+
| ``.inp`` | :class:`pyvista.AVSucdReader` |
+----------------+---------------------------------------------+
| ``.jpg`` | :class:`pyvista.JPEGReader` |
+----------------+---------------------------------------------+
| ``.jpeg`` | :class:`pyvista.JPEGReader` |
+----------------+---------------------------------------------+
| ``.hdr`` | :class:`pyvista.HDRReader` |
+----------------+---------------------------------------------+
| ``.mha`` | :class:`pyvista.MetaImageReader` |
+----------------+---------------------------------------------+
| ``.mhd`` | :class:`pyvista.MetaImageReader` |
+----------------+---------------------------------------------+
| ``.nek5000`` | :class:`pyvista.Nek5000Reader` |
+----------------+---------------------------------------------+
| ``.nii`` | :class:`pyvista.NIFTIReader` |
+----------------+---------------------------------------------+
| ``.nii.gz`` | :class:`pyvista.NIFTIReader` |
+----------------+---------------------------------------------+
| ``.nhdr`` | :class:`pyvista.NRRDReader` |
+----------------+---------------------------------------------+
| ``.nrrd`` | :class:`pyvista.NRRDReader` |
+----------------+---------------------------------------------+
| ``.obj`` | :class:`pyvista.OBJReader` |
+----------------+---------------------------------------------+
| ``.p3d`` | :class:`pyvista.Plot3DMetaReader` |
+----------------+---------------------------------------------+
| ``.ply`` | :class:`pyvista.PLYReader` |
+----------------+---------------------------------------------+
| ``.png`` | :class:`pyvista.PNGReader` |
+----------------+---------------------------------------------+
| ``.pnm`` | :class:`pyvista.PNMReader` |
+----------------+---------------------------------------------+
| ``.pts`` | :class:`pyvista.PTSReader` |
+----------------+---------------------------------------------+
| ``.pvd`` | :class:`pyvista.PVDReader` |
+----------------+---------------------------------------------+
| ``.pvti`` | :class:`pyvista.XMLPImageDataReader` |
+----------------+---------------------------------------------+
| ``.pvtk`` | :class:`pyvista.VTKPDataSetReader` |
+----------------+---------------------------------------------+
| ``.pvtr`` | :class:`pyvista.XMLPRectilinearGridReader` |
+----------------+---------------------------------------------+
| ``.pvtu`` | :class:`pyvista.XMLPUnstructuredGridReader` |
+----------------+---------------------------------------------+
| ``.res`` | :class:`pyvista.MFIXReader` |
+----------------+---------------------------------------------+
| ``.segy`` | :class:`pyvista.SegYReader` |
+----------------+---------------------------------------------+
| ``.sgy`` | :class:`pyvista.SegYReader` |
+----------------+---------------------------------------------+
| ``.slc`` | :class:`pyvista.SLCReader` |
+----------------+---------------------------------------------+
| ``.stl`` | :class:`pyvista.STLReader` |
+----------------+---------------------------------------------+
| ``.tif`` | :class:`pyvista.TIFFReader` |
+----------------+---------------------------------------------+
| ``.tiff`` | :class:`pyvista.TIFFReader` |
+----------------+---------------------------------------------+
| ``.tri`` | :class:`pyvista.BinaryMarchingCubesReader` |
+----------------+---------------------------------------------+
| ``.vrt`` | :class:`pyvista.ProStarReader` |
+----------------+---------------------------------------------+
| ``.vti`` | :class:`pyvista.XMLImageDataReader` |
+----------------+---------------------------------------------+
| ``.vtk`` | :class:`pyvista.VTKDataSetReader` |
+----------------+---------------------------------------------+
| ``.vtkhdf`` | :class:`pyvista.HDFReader` |
+----------------+---------------------------------------------+
| ``.vtm`` | :class:`pyvista.XMLMultiBlockDataReader` |
+----------------+---------------------------------------------+
| ``.vtmb`` | :class:`pyvista.XMLMultiBlockDataReader` |
+----------------+---------------------------------------------+
| ``.vtp`` | :class:`pyvista.XMLPolyDataReader` |
+----------------+---------------------------------------------+
| ``.vtr`` | :class:`pyvista.XMLRectilinearGridReader` |
+----------------+---------------------------------------------+
| ``.vts`` | :class:`pyvista.XMLStructuredGridReader` |
+----------------+---------------------------------------------+
| ``.vtu`` | :class:`pyvista.XMLUnstructuredGridReader` |
+----------------+---------------------------------------------+
| ``.xdmf`` | :class:`pyvista.XdmfReader` |
+----------------+---------------------------------------------+
| ``.vtpd`` | :class:`pyvista.XMLPartitionedDataSetReader`|
+----------------+---------------------------------------------+
Parameters
----------
filename : str, Path
The string path to the file to read.
force_ext : str, optional
An extension to force a specific reader to be chosen.
Returns
-------
pyvista.BaseReader
A subclass of :class:`pyvista.BaseReader` is returned based on file type.
Examples
--------
>>> import pyvista as pv
>>> from pyvista import examples
>>> from pathlib import Path
>>> filename = examples.download_human(load=False)
>>> Path(filename).name
'Human.vtp'
>>> reader = pv.get_reader(filename)
>>> reader
XMLPolyDataReader('...Human.vtp')
>>> mesh = reader.read()
>>> mesh
PolyData ...
>>> mesh.plot(color='lightblue')
The following code block uses a built-in example file, displays an airplane mesh and returns the camera’s position:
# Get a sample file
filename = examples.planefile
filename
'/home/runner/.virtualenvs/.venv/lib/python3.12/site-packages/pyvista/examples/airplane.ply'
Note the above filename, it’s a .ply
file - one of the many supported
formats in PyVista.
Use pv.read
to load the file as a mesh:
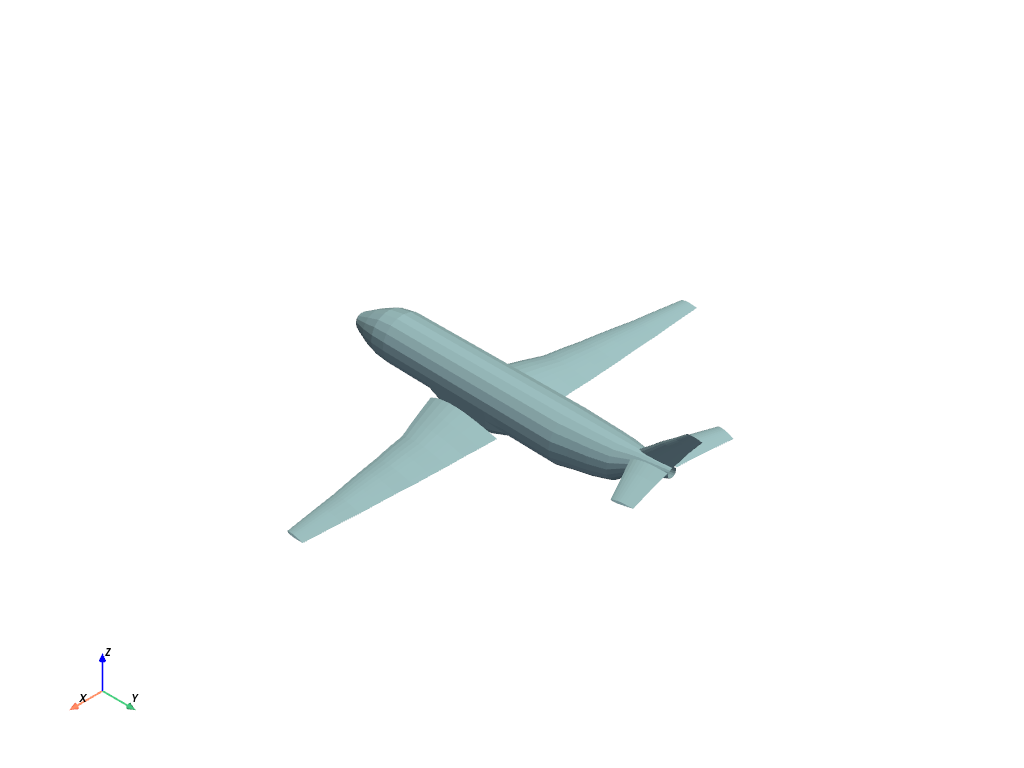
The points from the mesh are directly accessible as a NumPy array:
pyvista_ndarray([[896.994 , 48.7601 , 82.2656 ],
[906.593 , 48.7601 , 80.7452 ],
[907.539 , 55.4902 , 83.6581 ],
...,
[806.665 , 627.363 , 5.11482],
[806.665 , 654.432 , 7.51998],
[806.665 , 681.537 , 9.48744]],
shape=(1335, 3), dtype=float32)
The faces from the mesh are also directly accessible as a NumPy array:
mesh.faces.reshape(-1, 4)[:, 1:] # triangular faces
array([[ 0, 1, 2],
[ 0, 2, 3],
[ 4, 5, 1],
...,
[1324, 1333, 1323],
[1325, 1216, 1334],
[1325, 1334, 1324]], shape=(2452, 3))
Loading other files types is just as easy! Simply pass your file path to the
pyvista.read()
function and that’s it!
Here are a few other examples - simply replace examples.download_*
in the
examples below with pyvista.read('path/to/you/file.ext')
Example STL file:
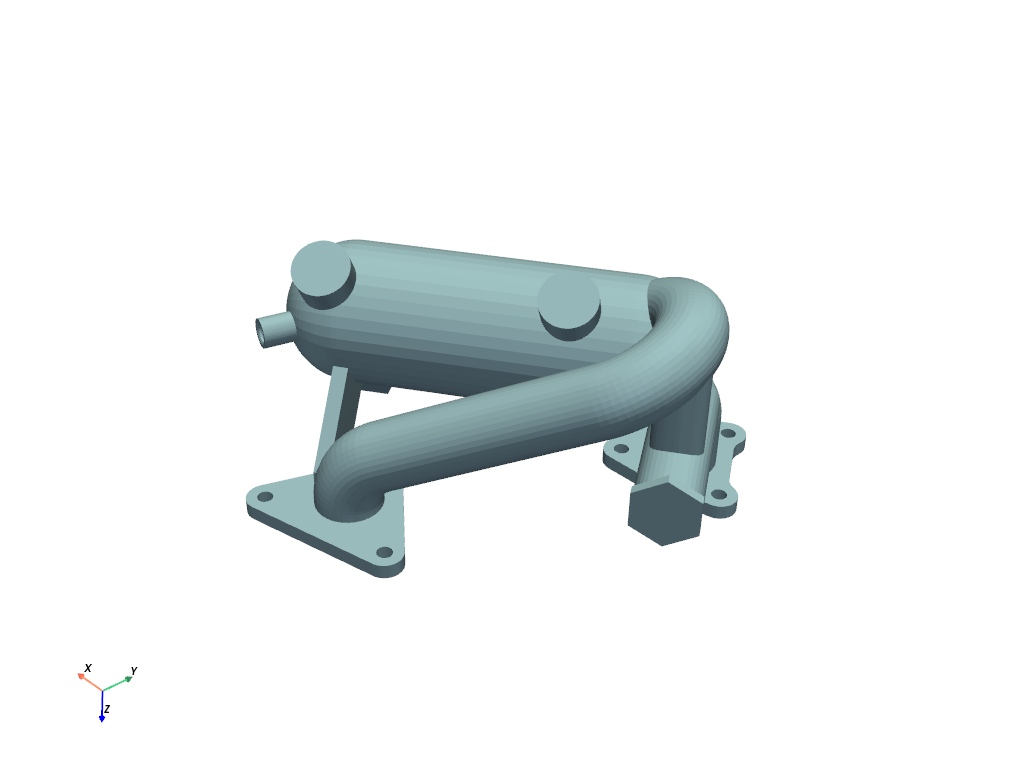
Example OBJ file
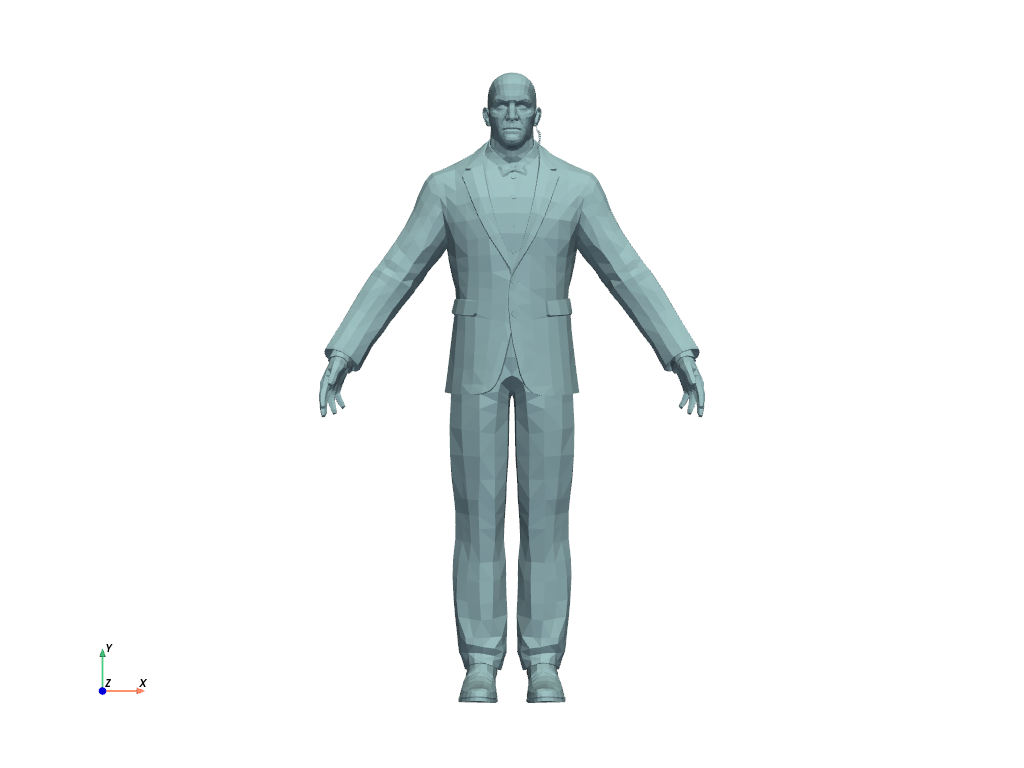
Example BYU file
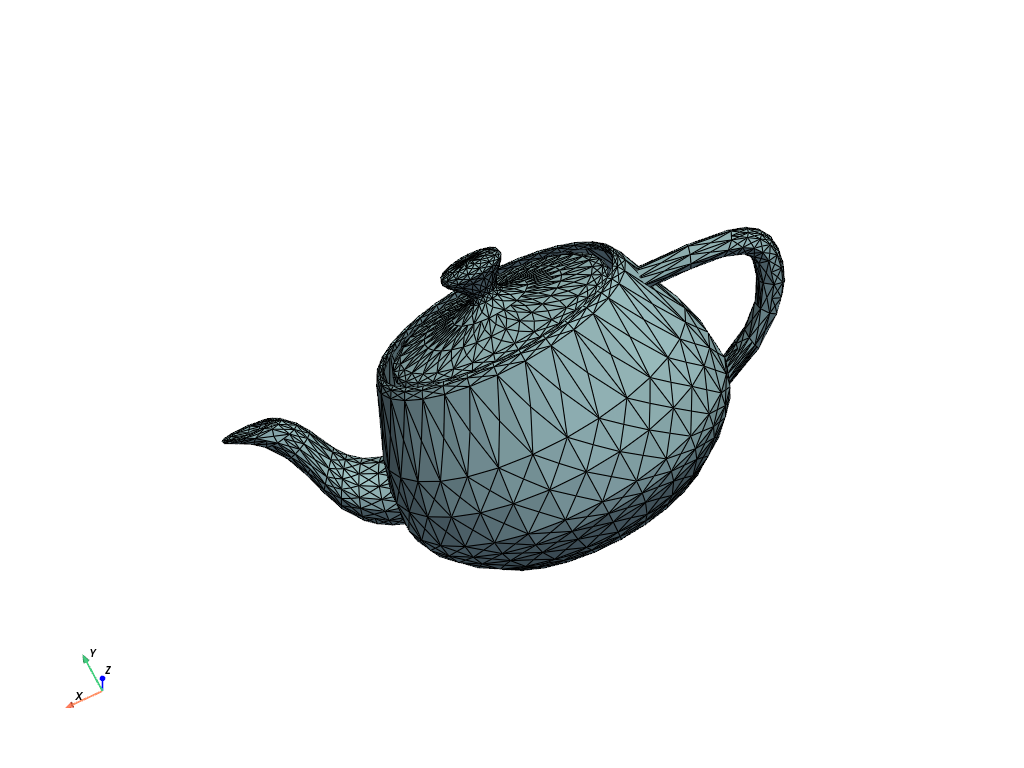
Example VTK file
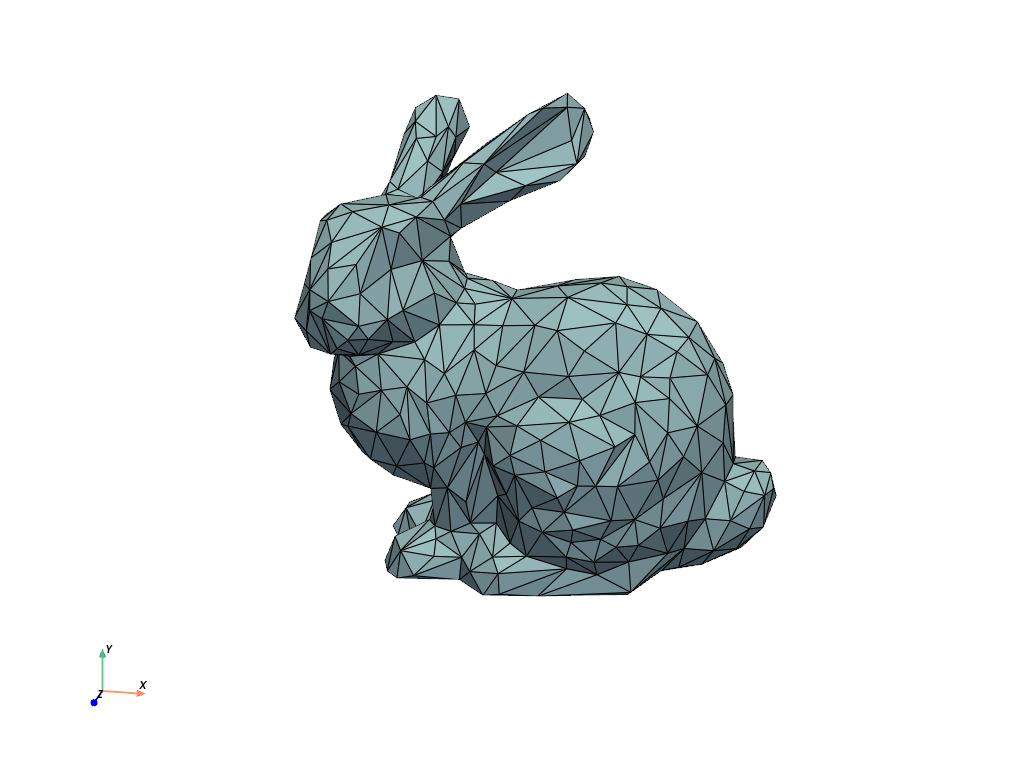
Exercise#
Read a file yourself with pyvista.read()
. If you have a supported file
format, use that! Otherwise, download this file:
pyvista/pyvista-tutorial
# (your code here)
# mesh = pv.read('path/to/file.vtk)
Total running time of the script: (0 minutes 4.060 seconds)